Here are 10 JavaScript tricks that aren’t just going to make your code more efficient, but they’ll also leave you thinking, “Why didn’t I know about this sooner?” These gems will empower you to code with confidence, efficiency, and flair. Let’s dive into this adventure!
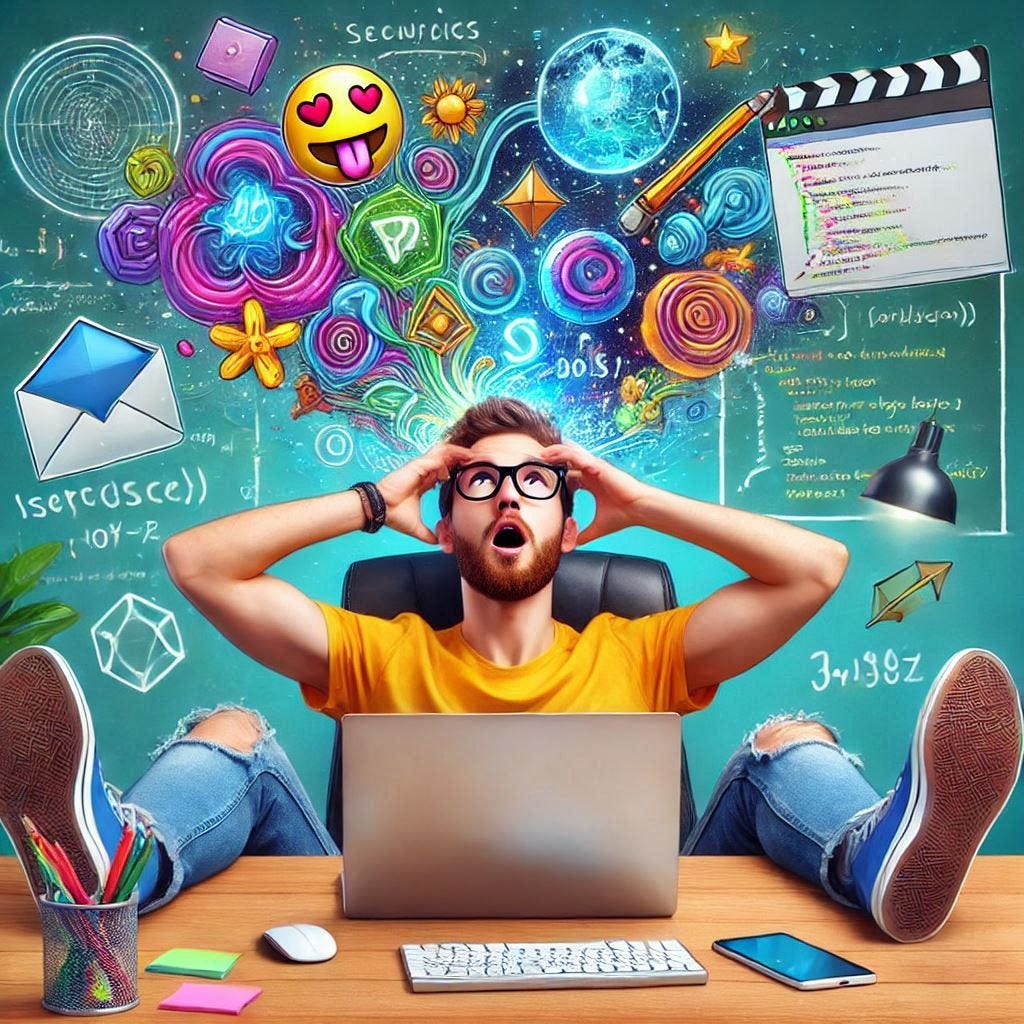
1. Template Literals: Your Code’s New Best Friend 🎩✨
Imagine being able to create dynamic strings that read like natural sentences. That’s the magic of template literals! No more ugly concatenation, no more errors from missing spaces. Just clean, elegant strings that make your code flow smoothly.
Example:
const name = 'Alice';
const age = 25;
const greeting = `Hey, I’m ${name}, and I’m ${age} years old. Cool, huh?`;
console.log(greeting); // "Hey, I’m Alice, and I’m 25 years old. Cool, huh?"
It’s like writing a sentence, not code, and it just feels so right. Plus, multi-line strings? No problem! Just hit “Enter” and keep typing:
const message = `Hello there!
I hope you're doing well.
Stay awesome!`;
console.log(message);
Magic? Absolutely.
Imagine being able to create dynamic strings that read like natural sentences. That’s the magic of template literals! No more ugly concatenation, no more errors from missing spaces. Just clean, elegant strings that make your code flow smoothly.
Example:
const name = 'Alice';
const age = 25;
const greeting = `Hey, I’m ${name}, and I’m ${age} years old. Cool, huh?`;
console.log(greeting); // "Hey, I’m Alice, and I’m 25 years old. Cool, huh?"
It’s like writing a sentence, not code, and it just feels so right. Plus, multi-line strings? No problem! Just hit “Enter” and keep typing:
const message = `Hello there!
I hope you're doing well.
Stay awesome!`;
console.log(message);
Magic? Absolutely.
2. Destructuring: Unpack the Power of Objects and Arrays 📦🔓
No more laboriously accessing each property with repetitive code. Destructuring lets you pull values from arrays and objects with ease, like unwrapping a gift. It’s not just efficient — it’s fun!
Array Example:
const [firstName, lastName] = ['John', 'Doe'];
console.log(firstName); // John
console.log(lastName); // Doe
Object Example:
const user = { name: 'Alice', age: 25 };
const { name, age } = user;
console.log(name); // Alice
console.log(age); // 25
Suddenly, everything falls into place with just a few lines of elegant code. 🎁
No more laboriously accessing each property with repetitive code. Destructuring lets you pull values from arrays and objects with ease, like unwrapping a gift. It’s not just efficient — it’s fun!
Array Example:
const [firstName, lastName] = ['John', 'Doe'];
console.log(firstName); // John
console.log(lastName); // Doe
Object Example:
const user = { name: 'Alice', age: 25 };
const { name, age } = user;
console.log(name); // Alice
console.log(age); // 25
Suddenly, everything falls into place with just a few lines of elegant code. 🎁
3. Default Parameters: Never Worry About Missing Arguments Again 🔧
We’ve all been there — passing arguments to functions and wondering, “What if I forget one?!” Enter default parameters, the safety net you didn’t know you needed. Now your functions can handle missing arguments like a pro.
Example:
function greet(name = 'Guest') {
console.log(`Hello, ${name}! Welcome!`);
}
greet(); // Hello, Guest! Welcome!
greet('Bob'); // Hello, Bob! Welcome!
It’s like having a backup plan that’s always there, just in case.
We’ve all been there — passing arguments to functions and wondering, “What if I forget one?!” Enter default parameters, the safety net you didn’t know you needed. Now your functions can handle missing arguments like a pro.
Example:
function greet(name = 'Guest') {
console.log(`Hello, ${name}! Welcome!`);
}
greet(); // Hello, Guest! Welcome!
greet('Bob'); // Hello, Bob! Welcome!
It’s like having a backup plan that’s always there, just in case.
4. Arrow Functions: The Swift, Sleek, and Powerful Sidekick ⚡️
There’s something magical about simplicity, right? Arrow functions give you just that: sleek, concise code that makes your functions look like they came straight out of a superhero comic. No need for the old function
keyword; just a quick arrow =>
and bam – you’re coding like a pro.
Example:
const add = (a, b) => a + b;
console.log(add(5, 7)); // 12
Short, sweet, and powerful. Arrow functions are a stylish tool that’ll transform your coding persona.
There’s something magical about simplicity, right? Arrow functions give you just that: sleek, concise code that makes your functions look like they came straight out of a superhero comic. No need for the old function
keyword; just a quick arrow =>
and bam – you’re coding like a pro.
Example:
const add = (a, b) => a + b;
console.log(add(5, 7)); // 12
Short, sweet, and powerful. Arrow functions are a stylish tool that’ll transform your coding persona.
5. The Spread Operator: Your Code’s Secret Weapon 🌈
Merging arrays and objects used to be a hassle. But now, with the spread operator, it’s as simple as a flick of the wrist. Copy, merge, combine — all without breaking a sweat. It’s like magic for your data.
Array Example:
const fruits = ['apple', 'banana'];
const moreFruits = [...fruits, 'orange', 'mango'];
console.log(moreFruits); // ['apple', 'banana', 'orange', 'mango']
Object Example:
const user = { name: 'Alice', age: 25 };
const updatedUser = { ...user, job: 'Developer' };
console.log(updatedUser); // { name: 'Alice', age: 25, job: 'Developer' }
It feels like you’re crafting something beautiful — effortlessly.
Merging arrays and objects used to be a hassle. But now, with the spread operator, it’s as simple as a flick of the wrist. Copy, merge, combine — all without breaking a sweat. It’s like magic for your data.
Array Example:
const fruits = ['apple', 'banana'];
const moreFruits = [...fruits, 'orange', 'mango'];
console.log(moreFruits); // ['apple', 'banana', 'orange', 'mango']
Object Example:
const user = { name: 'Alice', age: 25 };
const updatedUser = { ...user, job: 'Developer' };
console.log(updatedUser); // { name: 'Alice', age: 25, job: 'Developer' }
It feels like you’re crafting something beautiful — effortlessly.
6. Object Property Shorthand: The Art of Clean Code 🏷️
Less is more. Object property shorthand eliminates redundancy in a blink, making your code cleaner and easier to read. If the key name is the same as the variable name, just pass the variable — no need for repetition. It’s as clean as it gets.
Example:
const name = 'Alice';
const age = 25;
const user = { name, age };
console.log(user); // { name: 'Alice', age: 25 }
It’s like simplifying your life by removing the clutter.
Less is more. Object property shorthand eliminates redundancy in a blink, making your code cleaner and easier to read. If the key name is the same as the variable name, just pass the variable — no need for repetition. It’s as clean as it gets.
Example:
const name = 'Alice';
const age = 25;
const user = { name, age };
console.log(user); // { name: 'Alice', age: 25 }
It’s like simplifying your life by removing the clutter.
7. setTimeout
: Give Your Code Time to Breathe ⏳
Sometimes, you need to pause and reflect. setTimeout
lets you delay actions, giving you that precious moment of space.
Example:
setTimeout(() => {
console.log('Hello after 2 seconds!');
}, 2000); // 2000ms = 2 seconds
It’s like letting your code breathe, waiting for the right moment before it takes action. Sometimes timing is everything.
Sometimes, you need to pause and reflect. setTimeout
lets you delay actions, giving you that precious moment of space.
Example:
setTimeout(() => {
console.log('Hello after 2 seconds!');
}, 2000); // 2000ms = 2 seconds
It’s like letting your code breathe, waiting for the right moment before it takes action. Sometimes timing is everything.
8. Truthy and Falsy: JavaScript’s Hidden Logic 💡
The universe of truthy and falsy values in JavaScript is both vast and powerful. It lets you check conditions without needing to overcomplicate things. It’s like a secret door that lets you solve problems faster.
Example:
const value = 'Hello';
if (value) {
console.log('This is truthy!');
} else {
console.log('This is falsy!');
}
Truthy and falsy values are there to help you write smarter, cleaner conditions. Once you understand it, your code feels almost intuitive.
The universe of truthy and falsy values in JavaScript is both vast and powerful. It lets you check conditions without needing to overcomplicate things. It’s like a secret door that lets you solve problems faster.
Example:
const value = 'Hello';
if (value) {
console.log('This is truthy!');
} else {
console.log('This is falsy!');
}
Truthy and falsy values are there to help you write smarter, cleaner conditions. Once you understand it, your code feels almost intuitive.
9. Async/Await: Embrace the Simplicity of Asynchronous Code 🧙♂️
Forget callbacks. Forget promises. Async/await is the way to go. Writing asynchronous code that’s readable and clean has never been this easy. It’s like you’ve unlocked the wizardry of the coding world.
Example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.log('Oops, something went wrong!', error);
}
}
Async/await transforms your code into something that reads like a novel.
Forget callbacks. Forget promises. Async/await is the way to go. Writing asynchronous code that’s readable and clean has never been this easy. It’s like you’ve unlocked the wizardry of the coding world.
Example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.log('Oops, something went wrong!', error);
}
}
Async/await transforms your code into something that reads like a novel.
10. Optional Chaining: The Safety Net You Didn’t Know You Needed 🛡️
Ever wondered, “What if a property doesn’t exist?” No worries, optional chaining has got your back. With just a simple ?.
, you can avoid runtime errors and gracefully handle null
or undefined
.
Example:
const user = {
profile: {
name: 'Alice',
address: {
city: 'Wonderland',
},
},
};
console.log(user?.profile?.address?.city); // Wonderland
console.log(user?.profile?.phone); // undefined
Optional chaining is your shield against unexpected errors, allowing you to write safer, more robust code.
Ever wondered, “What if a property doesn’t exist?” No worries, optional chaining has got your back. With just a simple ?.
, you can avoid runtime errors and gracefully handle null
or undefined
.
Example:
const user = {
profile: {
name: 'Alice',
address: {
city: 'Wonderland',
},
},
};
console.log(user?.profile?.address?.city); // Wonderland
console.log(user?.profile?.phone); // undefined
Optional chaining is your shield against unexpected errors, allowing you to write safer, more robust code.
💥 Conclusion: A New Chapter in Your JavaScript Journey
JavaScript is full of hidden treasures, and now you’ve uncovered 10 of its most powerful tricks. Each one is a little step toward becoming a JavaScript master. Don’t just read these tips — try them out, experiment, and see how they transform your code.
With these tricks in your toolkit, you’ll not only code faster but with more creativity, confidence, and joy. Ready to make your code as brilliant as your ideas? 🚀✨ Happy coding, fellow developer!
JavaScript is full of hidden treasures, and now you’ve uncovered 10 of its most powerful tricks. Each one is a little step toward becoming a JavaScript master. Don’t just read these tips — try them out, experiment, and see how they transform your code.
With these tricks in your toolkit, you’ll not only code faster but with more creativity, confidence, and joy. Ready to make your code as brilliant as your ideas? 🚀✨ Happy coding, fellow developer!
1. Template Literals: Your Code’s New Best Friend 🎩✨
Imagine being able to create dynamic strings that read like natural sentences. That’s the magic of template literals! No more ugly concatenation, no more errors from missing spaces. Just clean, elegant strings that make your code flow smoothly.
Example:
const name = 'Alice';
const age = 25;
const greeting = `Hey, I’m ${name}, and I’m ${age} years old. Cool, huh?`;
console.log(greeting); // "Hey, I’m Alice, and I’m 25 years old. Cool, huh?"
It’s like writing a sentence, not code, and it just feels so right. Plus, multi-line strings? No problem! Just hit “Enter” and keep typing:
const message = `Hello there!
I hope you're doing well.
Stay awesome!`;
console.log(message);
Magic? Absolutely.
Imagine being able to create dynamic strings that read like natural sentences. That’s the magic of template literals! No more ugly concatenation, no more errors from missing spaces. Just clean, elegant strings that make your code flow smoothly.
Example:
const name = 'Alice';
const age = 25;
const greeting = `Hey, I’m ${name}, and I’m ${age} years old. Cool, huh?`;
console.log(greeting); // "Hey, I’m Alice, and I’m 25 years old. Cool, huh?"
It’s like writing a sentence, not code, and it just feels so right. Plus, multi-line strings? No problem! Just hit “Enter” and keep typing:
const message = `Hello there!
I hope you're doing well.
Stay awesome!`;
console.log(message);
Magic? Absolutely.
2. Destructuring: Unpack the Power of Objects and Arrays 📦🔓
No more laboriously accessing each property with repetitive code. Destructuring lets you pull values from arrays and objects with ease, like unwrapping a gift. It’s not just efficient — it’s fun!
Array Example:
const [firstName, lastName] = ['John', 'Doe'];
console.log(firstName); // John
console.log(lastName); // Doe
Object Example:
const user = { name: 'Alice', age: 25 };
const { name, age } = user;
console.log(name); // Alice
console.log(age); // 25
Suddenly, everything falls into place with just a few lines of elegant code. 🎁
No more laboriously accessing each property with repetitive code. Destructuring lets you pull values from arrays and objects with ease, like unwrapping a gift. It’s not just efficient — it’s fun!
Array Example:
const [firstName, lastName] = ['John', 'Doe'];
console.log(firstName); // John
console.log(lastName); // Doe
Object Example:
const user = { name: 'Alice', age: 25 };
const { name, age } = user;
console.log(name); // Alice
console.log(age); // 25
Suddenly, everything falls into place with just a few lines of elegant code. 🎁
3. Default Parameters: Never Worry About Missing Arguments Again 🔧
We’ve all been there — passing arguments to functions and wondering, “What if I forget one?!” Enter default parameters, the safety net you didn’t know you needed. Now your functions can handle missing arguments like a pro.
Example:
function greet(name = 'Guest') {
console.log(`Hello, ${name}! Welcome!`);
}
greet(); // Hello, Guest! Welcome!
greet('Bob'); // Hello, Bob! Welcome!
It’s like having a backup plan that’s always there, just in case.
We’ve all been there — passing arguments to functions and wondering, “What if I forget one?!” Enter default parameters, the safety net you didn’t know you needed. Now your functions can handle missing arguments like a pro.
Example:
function greet(name = 'Guest') {
console.log(`Hello, ${name}! Welcome!`);
}
greet(); // Hello, Guest! Welcome!
greet('Bob'); // Hello, Bob! Welcome!
It’s like having a backup plan that’s always there, just in case.
4. Arrow Functions: The Swift, Sleek, and Powerful Sidekick ⚡️
There’s something magical about simplicity, right? Arrow functions give you just that: sleek, concise code that makes your functions look like they came straight out of a superhero comic. No need for the old function
keyword; just a quick arrow =>
and bam – you’re coding like a pro.
Example:
const add = (a, b) => a + b;
console.log(add(5, 7)); // 12
Short, sweet, and powerful. Arrow functions are a stylish tool that’ll transform your coding persona.
There’s something magical about simplicity, right? Arrow functions give you just that: sleek, concise code that makes your functions look like they came straight out of a superhero comic. No need for the old function
keyword; just a quick arrow =>
and bam – you’re coding like a pro.
Example:
const add = (a, b) => a + b;
console.log(add(5, 7)); // 12
Short, sweet, and powerful. Arrow functions are a stylish tool that’ll transform your coding persona.
5. The Spread Operator: Your Code’s Secret Weapon 🌈
Merging arrays and objects used to be a hassle. But now, with the spread operator, it’s as simple as a flick of the wrist. Copy, merge, combine — all without breaking a sweat. It’s like magic for your data.
Array Example:
const fruits = ['apple', 'banana'];
const moreFruits = [...fruits, 'orange', 'mango'];
console.log(moreFruits); // ['apple', 'banana', 'orange', 'mango']
Object Example:
const user = { name: 'Alice', age: 25 };
const updatedUser = { ...user, job: 'Developer' };
console.log(updatedUser); // { name: 'Alice', age: 25, job: 'Developer' }
It feels like you’re crafting something beautiful — effortlessly.
Merging arrays and objects used to be a hassle. But now, with the spread operator, it’s as simple as a flick of the wrist. Copy, merge, combine — all without breaking a sweat. It’s like magic for your data.
Array Example:
const fruits = ['apple', 'banana'];
const moreFruits = [...fruits, 'orange', 'mango'];
console.log(moreFruits); // ['apple', 'banana', 'orange', 'mango']
Object Example:
const user = { name: 'Alice', age: 25 };
const updatedUser = { ...user, job: 'Developer' };
console.log(updatedUser); // { name: 'Alice', age: 25, job: 'Developer' }
It feels like you’re crafting something beautiful — effortlessly.
6. Object Property Shorthand: The Art of Clean Code 🏷️
Less is more. Object property shorthand eliminates redundancy in a blink, making your code cleaner and easier to read. If the key name is the same as the variable name, just pass the variable — no need for repetition. It’s as clean as it gets.
Example:
javascript
const name = 'Alice';
const age = 25;
const user = { name, age };
console.log(user); // { name: 'Alice', age: 25 }
It’s like simplifying your life by removing the clutter.
Less is more. Object property shorthand eliminates redundancy in a blink, making your code cleaner and easier to read. If the key name is the same as the variable name, just pass the variable — no need for repetition. It’s as clean as it gets.
Example:
javascript
const name = 'Alice';
const age = 25;
const user = { name, age };
console.log(user); // { name: 'Alice', age: 25 }
It’s like simplifying your life by removing the clutter.
7. setTimeout
: Give Your Code Time to Breathe ⏳
Sometimes, you need to pause and reflect. setTimeout
lets you delay actions, giving you that precious moment of space.
Example:
setTimeout(() => {
console.log('Hello after 2 seconds!');
}, 2000); // 2000ms = 2 seconds
It’s like letting your code breathe, waiting for the right moment before it takes action. Sometimes timing is everything.
Sometimes, you need to pause and reflect. setTimeout
lets you delay actions, giving you that precious moment of space.
Example:
setTimeout(() => {
console.log('Hello after 2 seconds!');
}, 2000); // 2000ms = 2 seconds
It’s like letting your code breathe, waiting for the right moment before it takes action. Sometimes timing is everything.
8. Truthy and Falsy: JavaScript’s Hidden Logic 💡
The universe of truthy and falsy values in JavaScript is both vast and powerful. It lets you check conditions without needing to overcomplicate things. It’s like a secret door that lets you solve problems faster.
Example:
const value = 'Hello';
if (value) {
console.log('This is truthy!');
} else {
console.log('This is falsy!');
}
Truthy and falsy values are there to help you write smarter, cleaner conditions. Once you understand it, your code feels almost intuitive.
The universe of truthy and falsy values in JavaScript is both vast and powerful. It lets you check conditions without needing to overcomplicate things. It’s like a secret door that lets you solve problems faster.
Example:
const value = 'Hello';
if (value) {
console.log('This is truthy!');
} else {
console.log('This is falsy!');
}
Truthy and falsy values are there to help you write smarter, cleaner conditions. Once you understand it, your code feels almost intuitive.
9. Async/Await: Embrace the Simplicity of Asynchronous Code 🧙♂️
Forget callbacks. Forget promises. Async/await is the way to go. Writing asynchronous code that’s readable and clean has never been this easy. It’s like you’ve unlocked the wizardry of the coding world.
Example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.log('Oops, something went wrong!', error);
}
}
Async/await transforms your code into something that reads like a novel.
Forget callbacks. Forget promises. Async/await is the way to go. Writing asynchronous code that’s readable and clean has never been this easy. It’s like you’ve unlocked the wizardry of the coding world.
Example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.log('Oops, something went wrong!', error);
}
}
Async/await transforms your code into something that reads like a novel.
10. Optional Chaining: The Safety Net You Didn’t Know You Needed 🛡️
Ever wondered, “What if a property doesn’t exist?” No worries, optional chaining has got your back. With just a simple ?.
, you can avoid runtime errors and gracefully handle null
or undefined
.
Example:
const user = {
profile: {
name: 'Alice',
address: {
city: 'Wonderland',
},
},
};
console.log(user?.profile?.address?.city); // Wonderland
console.log(user?.profile?.phone); // undefined
Optional chaining is your shield against unexpected errors, allowing you to write safer, more robust code.
Ever wondered, “What if a property doesn’t exist?” No worries, optional chaining has got your back. With just a simple ?.
, you can avoid runtime errors and gracefully handle null
or undefined
.
Example:
const user = {
profile: {
name: 'Alice',
address: {
city: 'Wonderland',
},
},
};
console.log(user?.profile?.address?.city); // Wonderland
console.log(user?.profile?.phone); // undefined
Optional chaining is your shield against unexpected errors, allowing you to write safer, more robust code.
💥 Conclusion: A New Chapter in Your JavaScript Journey
JavaScript is full of hidden treasures, and now you’ve uncovered 10 of its most powerful tricks. Each one is a little step toward becoming a JavaScript master. Don’t just read these tips — try them out, experiment, and see how they transform your code.
With these tricks in your toolkit, you’ll not only code faster but with more creativity, confidence, and joy. Ready to make your code as brilliant as your ideas? 🚀✨ Happy coding, fellow developer!
JavaScript is full of hidden treasures, and now you’ve uncovered 10 of its most powerful tricks. Each one is a little step toward becoming a JavaScript master. Don’t just read these tips — try them out, experiment, and see how they transform your code.
With these tricks in your toolkit, you’ll not only code faster but with more creativity, confidence, and joy. Ready to make your code as brilliant as your ideas? 🚀✨ Happy coding, fellow developer!
0 Comments